3. Pro Guide: Generate Advanced Collatz Networks
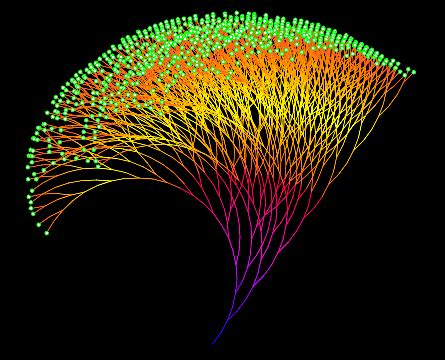
The Collatz Conjecture, also known as the 3n + 1 problem, is a fascinating mathematical concept that has intrigued mathematicians and enthusiasts alike for decades. This guide aims to delve into the advanced techniques and strategies for generating Collatz networks, offering a comprehensive understanding of this intriguing mathematical phenomenon.
Understanding the Collatz Conjecture
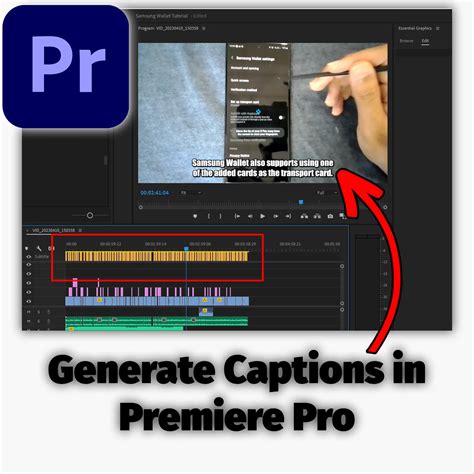
At its core, the Collatz Conjecture is a simple recursive function that operates on natural numbers. Given an initial number n, the function follows these rules:
- If n is even, divide it by 2.
- If n is odd, multiply it by 3 and add 1.
The process is repeated with the new value, and the sequence of numbers generated is known as the Collatz sequence or hailstone sequence. The conjecture posits that, regardless of the starting number, this sequence will always reach 1 eventually.
Visualizing Collatz Networks
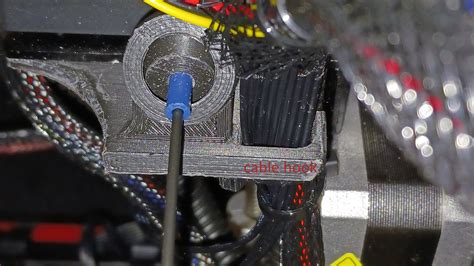
To gain deeper insights into the Collatz Conjecture, we can visualize it as a network or graph. Each number in the sequence becomes a node, and the arrows represent the transformation rules. For instance, an even number n is connected to n/2, while an odd number n is connected to 3n + 1.
Here's a simple example of a Collatz network for the starting number 6:
6 | → | 3 | → | 10 | → | 5 | → | 16 | → | 8 | → | 4 | → | 2 | → | 1 |
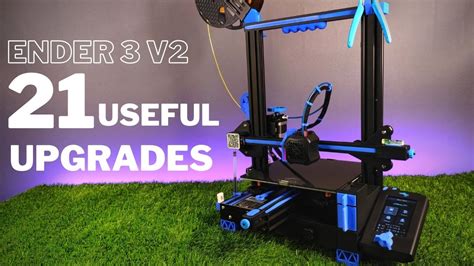
As you can see, the sequence eventually reaches 1, supporting the Collatz Conjecture.
Generating Collatz Networks

To generate Collatz networks efficiently, we can utilize various programming languages and tools. Here's a step-by-step guide using Python:
Step 1: Install Required Libraries
First, ensure you have Python installed on your system. Then, open your preferred code editor and install the necessary libraries:
pip install networkx matplotlib
Step 2: Import Libraries and Define Functions
Import the required libraries and define functions to calculate the next number in the sequence and create the network:
import networkx as nx
import matplotlib.pyplot as plt
def next_number(n):
if n % 2 == 0:
return n / 2
else:
return 3 * n + 1
def create_network(start_number, max_depth=10):
G = nx.DiGraph()
G.add_node(start_number)
current_number = start_number
for depth in range(1, max_depth + 1):
next_number_value = next_number(current_number)
G.add_node(next_number_value)
G.add_edge(current_number, next_number_value)
current_number = next_number_value
return G
Step 3: Generate and Visualize the Network
Call the create_network
function with your desired starting number and maximum depth. Then, visualize the network using networkx
and matplotlib
:
start_number = 6
max_depth = 10
G = create_network(start_number, max_depth)
plt.figure(figsize=(10, 8))
pos = nx.spring_layout(G)
nx.draw(G, pos, with_labels=True, node_size=600, node_color='lightblue', edge_color='gray')
nx.draw_networkx_edge_labels(G, pos)
plt.show()
This code will generate and display a Collatz network for the starting number 6 up to a depth of 10.
Advanced Techniques
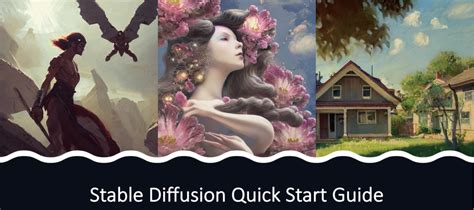
1. Optimizing Network Generation
To improve the efficiency of network generation, you can optimize the code by implementing memoization. This technique stores the results of expensive function calls and returns the cached result when the same inputs occur again. Here's how you can modify the next_number
function:
memo = {}
def next_number(n):
if n in memo:
return memo[n]
if n % 2 == 0:
result = n / 2
else:
result = 3 * n + 1
memo[n] = result
return result
🌟 Note: Memoization can significantly speed up network generation, especially for larger starting numbers and deeper networks.
2. Visualizing Large Networks
As you explore larger starting numbers and deeper networks, the visualization can become cluttered. To enhance readability, you can adjust the layout and styling:
pos = nx.spring_layout(G, k=0.5) # Adjust k for better spacing
nx.draw(G, pos, with_labels=True, node_size=500, node_color='lightgray', edge_color='gray', font_size=8)
nx.draw_networkx_edge_labels(G, pos, font_size=6)
Additionally, you can save the network as an image file using plt.savefig('collatz_network.png')
to share or analyze it further.
3. Exploring Patterns and Properties
While the Collatz Conjecture remains unproven, exploring patterns and properties within Collatz networks can provide valuable insights. Here are a few observations:
- The sequence often exhibits a "zig-zag" pattern, alternating between even and odd numbers.
- Certain numbers, known as supernumbers, have the property that their Collatz sequences eventually reach a cycle of length 2.
- The average number of steps to reach 1 seems to converge to a constant value as the starting number increases.
Conclusion
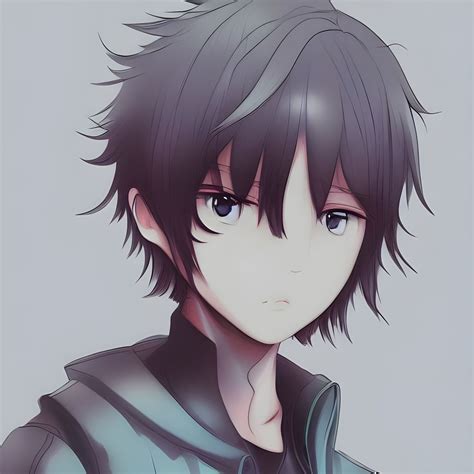
Generating Collatz networks offers a captivating journey into the world of mathematics and graph theory. By visualizing and analyzing these networks, we gain deeper insights into the behavior of the Collatz Conjecture. While the conjecture remains a mystery, the process of exploration and discovery is what makes mathematics so captivating.
FAQ

What is the Collatz Conjecture?
+The Collatz Conjecture is a mathematical hypothesis stating that, regardless of the starting number, the Collatz sequence will always reach 1.
How can I generate Collatz networks efficiently?
+Utilize programming languages like Python and libraries such as networkx
and matplotlib
to generate and visualize Collatz networks efficiently.
What are some advanced techniques for Collatz network generation?
+Techniques like memoization can optimize network generation, while adjusting layout and styling can enhance the visualization of large networks.
Are there any patterns or properties in Collatz networks?
+Yes, Collatz networks exhibit patterns like the “zig-zag” sequence and have certain numbers, called supernumbers, that reach a cycle of length 2.