15+ Excel Python Tips: The Ultimate Cell Reference Guide

Mastering cell references in Excel with Python is a powerful skill for data manipulation and analysis. This guide offers a comprehensive overview of cell referencing techniques, ensuring your Python-Excel workflow is efficient and accurate. From basic to advanced referencing, we'll cover it all, making your Excel data handling a breeze.
Understanding Cell References in Excel with Python

Cell references are the backbone of Excel, allowing you to work with data efficiently. When integrating Python, these references become even more crucial for data manipulation and analysis. Understanding how to reference cells accurately is key to unlocking Excel's full potential with Python.
Basic Cell References

Start with the fundamentals. Basic cell references in Excel are straightforward and essential for any data manipulation task. They are the building blocks for more complex referencing and formulae.
Absolute References ($)

Absolute references are fixed, meaning the cell they refer to doesn't change when copied or filled. Use the dollar sign ($) to create an absolute reference. For instance, $A$1
always refers to the cell in column A and row 1, regardless of where the formula is copied.
Relative References (No $)
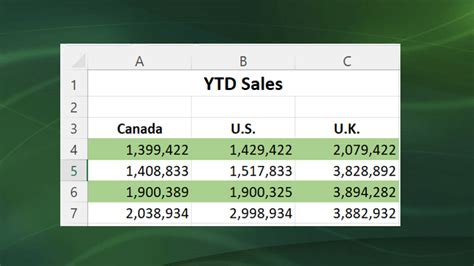
Relative references, on the other hand, change when copied or filled. They're dynamic, adjusting to the new cell's position. For example, if you copy a formula with a relative reference from cell B2
to C3
, the reference will update to C3
, ensuring the formula operates on the correct cell.
Advanced Cell References

Once you've grasped the basics, it's time to explore more advanced cell referencing techniques. These methods enhance your data manipulation capabilities, making complex tasks simpler and more efficient.
Mixed References

Mixed references combine absolute and relative references. They're useful when you want to fix one part of a reference while allowing the other to change. For instance, $A2
fixes the column reference (A) while allowing the row reference (2) to change.
3D References

3D references enable you to work with multiple sheets simultaneously. They're especially handy when you need to perform the same operation on different sheets. Simply include the sheet names separated by an exclamation mark (!), like Sheet1!A1:B5
, to reference a range of cells across multiple sheets.
External References

External references let you reference cells from other Excel workbooks. This is useful when you need to integrate data from multiple files. Simply use the workbook name followed by an exclamation mark (!) and the cell reference, e.g., [Book2.xlsx]Sheet1!A1
.
Referencing Cells with Python

Now, let's delve into how to reference cells in Excel using Python. The openpyxl
library is a powerful tool for this task, offering a seamless way to interact with Excel files.
Importing the Library

Begin by importing the openpyxl
library. This library provides functions to read, write, and manipulate Excel files in Python.
import openpyxl
Opening an Excel File
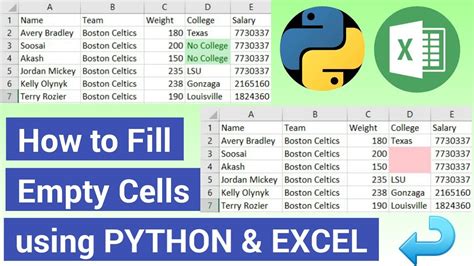
Use the load_workbook
function to open an existing Excel file. This function returns a Workbook
object, which you can use to access individual sheets and cells.
wb = openpyxl.load_workbook('your_file.xlsx')
Accessing Sheets

Sheets in an Excel workbook are accessible through the Workbook
object. You can retrieve a specific sheet using its name or index.
sheet = wb['Sheet1'] # Access by name
sheet = wb.active # Access the active sheet
sheet = wb[0] # Access by index (first sheet)
Reading Cell Values

To read the value of a cell, use the cell
attribute of the sheet object. Provide the cell's coordinates (e.g., 'A1'
) as an argument.
value = sheet['A1'].value
Writing to Cells

Writing to cells is just as easy. Simply assign a value to the value
attribute of the cell object.
sheet['A1'].value = 'Hello, Excel!'
Using Cell Ranges
You can also work with ranges of cells. The openpyxl
library provides a range
function to iterate over a range of cells.
for cell in sheet['A1':'C5']:
print(cell.value)
Tips and Tricks for Efficient Cell Referencing

Mastering cell referencing in Excel with Python takes practice. Here are some tips and tricks to make your workflow more efficient and accurate.
Use Named Ranges
Named ranges are a powerful way to reference cells or ranges of cells. They make your code more readable and maintainable. You can define named ranges in Excel and access them directly from Python using the openpyxl
library.
Handle Errors Gracefully
When referencing cells, errors can occur if a cell is empty or doesn't exist. Use try-except blocks to handle these errors gracefully and prevent your code from crashing.
Optimize with Caching
If you're working with large datasets, caching cell values can significantly improve performance. Instead of reading cell values directly from Excel, store them in a dictionary or list for faster access.
Utilize Excel Formulas
Don't reinvent the wheel. Excel has a vast array of built-in formulas for common tasks. Leverage these formulas in your Python code to simplify your data manipulation tasks.
Real-World Examples
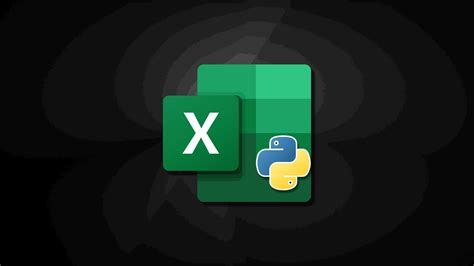
Let's put our knowledge into practice with some real-world examples. These scenarios will showcase how to apply cell referencing techniques in practical situations.
Data Cleaning
Clean and prepare data for analysis by removing duplicates, handling missing values, and standardizing formats. Cell referencing is crucial for identifying and manipulating specific data points.
Financial Analysis
Perform financial calculations and modeling by referencing cell values that represent financial metrics, such as revenue, expenses, and profits. Advanced cell referencing techniques can simplify complex financial models.
Data Visualization
Create visually appealing charts and graphs by referencing data ranges. Mixed references can be particularly useful when creating dynamic charts that update based on user input.
Conclusion: Unlocking Excel's Potential with Python

Cell referencing is a powerful tool in your Excel-Python toolkit. By understanding and utilizing these techniques, you can manipulate and analyze data with ease. Whether you're a data analyst, scientist, or engineer, mastering cell references will enhance your data-driven projects.
Remember, practice makes perfect. Experiment with different referencing techniques, explore the openpyxl
library's capabilities, and don't be afraid to ask for help. The Excel-Python community is vast and supportive, ready to assist you on your data journey.
FAQ

How do I create a named range in Excel?
+To create a named range in Excel, select the range of cells you want to name, then go to the Formulas tab and click on "Define Name." Enter a name for your range and click OK. Named ranges are a great way to improve the readability and maintainability of your Excel-Python code.
Can I use cell references in Excel formulas with Python?
+Absolutely! You can use cell references in Excel formulas just like you would in a regular Excel worksheet. The openpyxl
library provides functions to read and write cell values, allowing you to create and manipulate formulas directly from Python.
What's the best way to handle large datasets in Excel with Python?
+When working with large datasets, it's crucial to optimize your code for performance. Caching cell values, using named ranges, and handling errors gracefully can significantly improve the speed and stability of your Excel-Python workflow. Additionally, consider using Excel's built-in functions for common tasks to reduce the computational load on your Python code.
🌟 Note: Always ensure your Excel files are properly formatted and free of errors before attempting complex data manipulation tasks with Python. Regularly back up your data and test your code thoroughly to avoid unexpected issues.